Check if a value exists in array in Javascript
Check if a value exists in array in Javascript
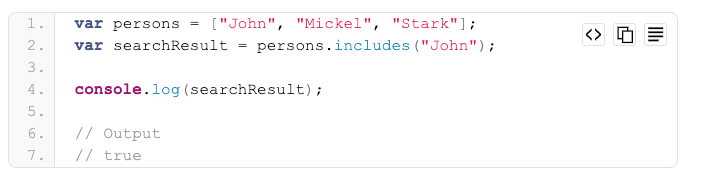
In this chapter, you will learn about how to check if a value exists in an array in Javascript. When you have an array of the elements, and you need to check whether the certain value exists or not.
Solution 1:
includes(): We can use an includes(), which is an array method and return a boolean value either true or false. If the value exists returns true otherwise it returns false. Let’s write an example of the same.
var persons = ["John", "Mickel", "Stark"]; var searchResult = persons.includes("John"); console.log(searchResult); // Output // true
The content of the array can be anything like string or numbers.
var gameScore = [100,10,89,60,50]; var searchResult = gameScore.includes(60); console.log(searchResult); // Output // true
Solution 2:
indexOf(): You can also use the method indexOf(), which returns the first index at which a given element can be found in the array, otherwise -1. let’s write an example for the same
var gameScore = [100,10,89,60,50]; var searchResult = gameScore.indexOf(60); console.log(searchResult); // Output // 3 //Try with number which doesnot exists var gameScore = [100,10,89,60,50]; var searchResult = gameScore.indexOf(67); console.log(searchResult); // Output // -1
Solution 3:
We can also use the for loop, with the matching condition, let’s write an example for the same
var gameScore = [100,10,89,60,50]; var depth = gameScore.length; while (depth--) { if (gameScore[depth] === 100) { console.log('The value exist...'); } } // Output // The value exist...