Comparison operators in python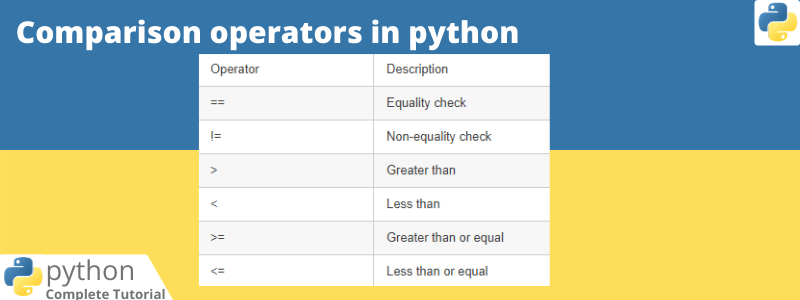
In this chapter, we will learn about comparison operators in python. Now let’s list the comparison operators
Operator | Description |
== | Equality check |
!= | Non-equality check |
> | Greater than |
< | Less than |
>= | Greater than or equal |
<= | Less than or equal |
Equality check:
The Equality check operator can be used to check whether two numbers are equal, also to check whether the two strings are equal. Let’s write an example for the same
Code snippet for checking whether two number are equal if 2 == 2: print('Numbers are equal') else: print('Numbers are not equal') # output # Numbers are equal
Non-equality check:
The Equality check operator can be used to check whether two numbers are not equal, also to check whether the two strings are not equal. Let’s write an example for the same
Code snippet for whether two number are not equal
if 1 != 2: print('Numbers are not equal') else: print('Numbers are equal') # output # Numbers are not equal
Greater than:
Greater than the operator can be used to check whether the left side operand is greater. Let’s write an example for the same
if 4 > 2: print('Number is greater') else: print('Number is not greater') # output # Number is greater
Less than:
Greater than the operator can be used to check whether the left side operand is greater. Let’s write an example for the same
if 2 < 4: print('Number is lesser') else: print('Number is not lesser') # output # Number is lesser
Greater than or equal:
Greater than or equal operator can be used to check whether the numbers or strings greater or equal. Let’s write an example for the same
if 2 >= 4: print('Number is greater or equal') else: print('Number is not lesser') # output # Number is not greater
Less than or equal:
Less than or equal operator can be used to check whether the numbers or strings less or equal. Let’s write an example for the same
if 4 <= 5: print('Number is lesser or equal') else: print('Number is not lesser') # output # Number is lesser or equal
One thought on “Comparison operators in python”
Comments are closed.