List in python with examples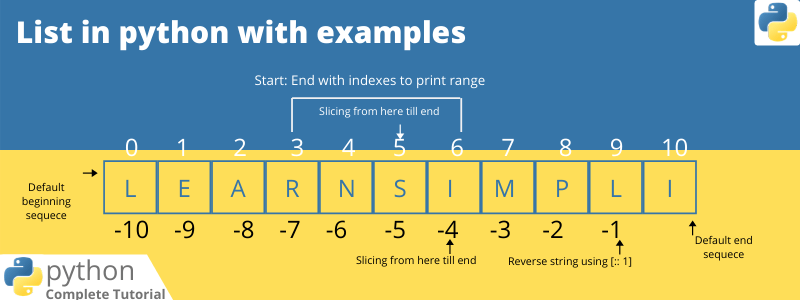
In this chapter, you will learn about the list in python with examples. As we studied in the chapter Data types in python, the list is one of the data types in the python
Lists:
The lists data type is similar to the javascript array. The list can be defined with the square brackets and values can be grouped with the comma. The following points can help you to understand the lists in a better way
- Lists can be indexed and sliced
- Lists can hold items of different types or similar types
- Lists also support operations like concatenation
- Lists are a mutable
- Lists can be nested
Python lists are very flexible to hold the data, let’s see examples
employeeDeail = ['John', 'Software developer', 5] print(len(employeeDeail)) # output # 3
The list in python supports slicing and indexing, let’s see the example
studentName = ['John', 'Stark', 'Clark'] print(studentName[2]) print(studentName[1:]) # output # Clark # ['Stark', 'Clark']
The advantage of lists in python is that it supports concatenation, let’s write an example for concatenating two lists in the python
studentName = ['John', 'Stark', 'Clark'] studentName2 = ['Mickel', 'Darshan'] allStudent = studentName + studentName2 print(allStudent) # output # ['John', 'Stark', 'Clark', 'Mickel', 'Darshan']
The lists are mutable:
Now we will update the student name of john with some other name.
studentName = ['John', 'Stark', 'Clark'] print('************* Student old name ************') print(studentName[0]) # Update john name studentName[0] = 'Johns updated name' print('************* Student updated name **********') print(studentName[0]) # output # ************* Student old name ************ # John # ************* Student updated name ********** # Johns updated name
How can we add an element at the end of the list in python?
You can add the new element to the list with help of the append method, let’s write an example for the same
studentName = ['John', 'Stark', 'Clark'] print('************* Student names ************') print(studentName) # append the new element studentName.append('Mickel') print(studentName) # output # ************* Student names ************ # ['John', 'Stark', 'Clark'] # ['John', 'Stark', 'Clark', 'Mickel']
How can we remove an element from the end of the list in python?
You can remove an element from the end of a list with the help of the pop method, which removes the last element in the list.
studentName = ['John', 'Stark', 'Clark'] print('************* Student names ************') print(studentName) # pop an element studentName.pop() print(studentName) # output # ************* Student names ************ # ['John', 'Stark', 'Clark'] # ['John', 'Stark']
The pop method always returns a removed element, let’s see an example
studentName = ['John', 'Stark', 'Clark'] print('************* Student names ************') print(studentName) # append the new element removedName = studentName.pop() print('Removed element :'+removedName) print(studentName) # output # ************* Student names ************ # ['John', 'Stark', 'Clark'] # Removed element :Clark # ['John', 'Stark']
How can we remove a specific element?
You can remove the particular element in the list with help of element index, let see an example for the same
studentName = ['John', 'Stark', 'Clark'] print('************* Student names ************') print(studentName) # remove specific element removedName = studentName.pop(1) print('Removed element :'+removedName) print(studentName) # output # ************* Student names ************ # ['John', 'Stark', 'Clark'] # Removed element :Stark # ['John', 'Clark']
Sorting the list:
You can sort the list with the method sort(), note that the sort method doesn’t return the new list of the element, instead it updates the existing list
studentMarks = [90,20,10,50,4] print('************* Student marks ************') print(studentMarks) # now lets sort an array studentMarks.sort() print('************* Sorted Student marks ************') print(studentMarks) # output # ************* Student marks ************ # [90, 20, 10, 50, 4] # ************* Sorted Student marks ************ # [4, 10, 20, 50, 90]
You can also sort alphabets not only numbers
alphabets = ['d','e','a','d','c','s'] print('************* alphabets ************') print(alphabets) # now lets sort an array alphabets.sort() print('************* Sorted alphabets ************') print(alphabets) # output # ************* alphabets ************ # ['d', 'e', 'a', 'd', 'c', 's'] # ************* Sorted alphabets ************ # ['a', 'c', 'd', 'd', 'e', 's']
One thought on “List in python with examples”
Comments are closed.